
本文共 13266 字,大约阅读时间需要 44 分钟。
PHP中用MVC模式来实现数据库的增删改查
视图View
代表用户交互的页面、可以包含HTML界面、Smarty模板等和界面相关的元素。MVC设计模式对于视图的处理仅限于视图上数据的采集和处理,以及用户的点击、拖动等事件的处理,而不包括在视图上的业务流程处理。业务流程会交给模型层(Model)处理
模型Model
模型层是对业务流程、状态的处理以及业务规则的指定。业务流程的处理过程对其他层来说是黑箱操作,模型接受视图的请求处理数据,返回最终的处理结果。业务模型还有一个很重要的模型–数据模型,数据模型主要指实体对象的数据保存(持久化)。比如将一张订单保存到数据库,从数据库获取订单,所有和数据库相关的操作限定在该模型中。
控制器Controller
控制层是View层和Model层之间的一个桥梁,接收到用户的请求,将模型和视图匹配在一起,共同完成用户的请求。比如,用户点击一个链接,控制层接收到请求后,把信息传递给模型层,模型层处理完成之后返回视图给用户。
题目要求:
用MVC模式实现以下功能
-
首页导航栏中内置功能
-
查看数据库
-
点击Edit修改数据库内容
-
点击Delete后删除数据库内此记录,返回首页输出删除成功。
-
向数据库里增加数据
-
向搜索框输入查询的关键字
之后输出查询结果:
目录结构:
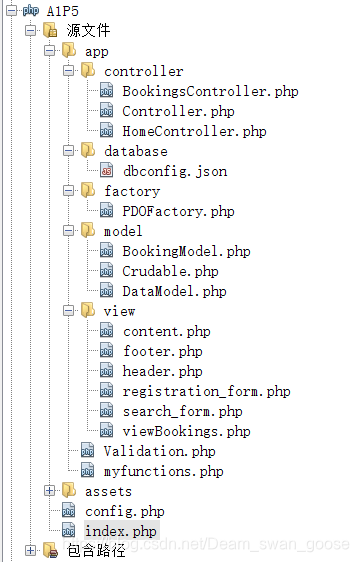
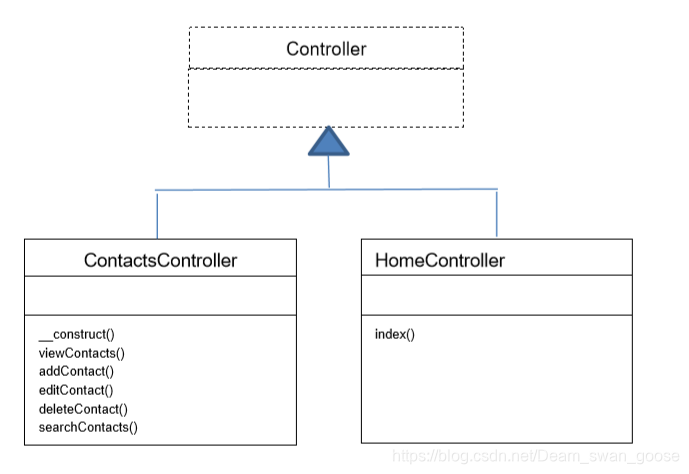
'alpha_spaces|true', 'lastname' => 'alpha_spaces|true', 'email' => 'email|true', 'mobile' => 'digits|true', 'photo' => 'photo|true', ]; //创建对象 function __construct() { $this->booking = new BookingModel(); } function index() { include_once 'view/content.php'; } function addBooking() { if (isset($_POST['submit'])) { $_POST['photo'] = $_FILES['photo']['name']; $values = [$_POST['firstname'], $_POST['lastname'], $_POST['email'], $_POST['mobile'], $_FILES['photo']['name']]; $validation = new Validation(); $errors = $validation->validate_form_data($_POST, $this->fields); if (count($errors) == 0) { $success = $this->booking->insertRecord($values); $filename = $_FILES["photo"]["name"]; $temp_file = $_FILES["photo"]["tmp_name"]; $destination = "assets/img/photos/$filename"; move_uploaded_file($temp_file, $destination); if ($success) { include_once "index.php"; } else { echo "Insert ERROR!"; } } else { include_once 'view/registration_form.php'; } } else { include_once 'view/registration_form.php'; } } function deleteBooking() { $id = $_GET['id']; $success = $this->booking->deleteRecord($id); if (!$success) { echo "Delete Failed!"; } else { $records = $this->booking->readRecords(); include_once("view/viewBookings.php"); } return $success; } function editBooking() { $id = $_GET['id']; $records = $this->booking->readRecords(); for ($i = 0; $i < count($records); $i++) { if ($records[$i]['id'] == $id) { $firstname_temp = $records[$i]['first_name']; $lastname_temp = $records[$i]['last_name']; $email_temp = $records[$i]['email']; $mobile_temp = $records[$i]['mobile']; } } if (isset($_POST['submit'])) { $_POST['photo'] = $_FILES['photo']['name']; $validation = new Validation(); $errors = $validation->validate_form_data($_POST, $this->fields); if (count($errors) == 0) { $values = [$_POST['firstname'], $_POST['lastname'], $_POST['email'], $_POST['mobile'], $_FILES['photo']['name']]; $filename = $_FILES["photo"]["name"]; $temp_file = $_FILES["photo"]["tmp_name"]; $destination = "assets/img/photos/$filename"; move_uploaded_file($temp_file, $destination); $success = $this->booking->updateRecord($id, $values); if ($success) { $firstname_temp = ""; $lastname_temp = ""; $email_temp = ""; $mobile_temp = ""; $photo_temp = ""; $records = $this->booking->readRecords(); include_once("view/viewBookings.php"); } else { echo "Update Failed!"; } } else { include_once 'view/registration_form.php'; } } else { include_once 'view/registration_form.php'; } } function searchBookings() { if (isset($_POST['Search'])) { $keyword = $_POST['search']; $search_record = $this->booking->searchRecord($keyword); if (isset($search_record)) { $records = $search_record; include_once 'view/viewBookings.php'; } else { echo "None"; } } else { include_once 'view/search_form.php'; } } function viewBookings() { $records = $this->booking->readRecords(); include_once 'view/viewBookings.php'; }}
app/controller/Controller.php
app/controller/HomeController.php
app/database/dbconfig.json
{ "DSN":"mysql:host=localhost:3306;dbname=contactsdb","USERNAME":"root","PASSWORD":""}
app/factory/PDOFactory.php
DSN; $username = $json_data->USERNAME; $password = $json_data->PASSWORD; $conn = new PDO($dsn, $username, $password); return $conn; }}
statement = $this->conn->query($sql); $this->statement->setFetchMode(PDO::FETCH_ASSOC); $records = $this->statement->fetchAll(); return $records; } function deleteRecord($id) { $sql = "delete from contacts where id=$id"; $this->statement = $this->conn->query($sql); $success = $this->statement->execute(); return $success; } function insertRecord($values) { $sql = 'insert into contacts ( first_name, last_name, email, mobile, photo_filename) values(?,?,?,?,?)'; $this->statement = $this->conn->prepare($sql); $success = $this->statement->execute($values); return $success; } function updateRecord($id, $values) { $sql = "update contacts set first_name=?, last_name=?, email=?, mobile=?, photo_filename=? where id=$id"; $this->statement = $this->conn->prepare($sql); $success = $this->statement->execute($values); return $success; } function searchRecord($keyword) { $sql = "select * from contacts where first_name like '%$keyword%' or last_name like '%$keyword%' or email like '%$keyword%' or mobile like '%$keyword%' or photo_filename like '%$keyword%'"; $this->statement = $this->conn->query($sql); $this->statement->setFetchMode(PDO::FETCH_ASSOC); $booking_records = $this->statement->fetchAll(); return $booking_records; }}
app/model/Crudable.php
app/model/DataModel.php
conn = PDOFactory::getConnection(); } function close() { $this->conn = null; }}
app/view/content.php
CONTACTS DATABASE
I AM A STUDENT IN THE WEB APPLICATION & SERVER MANAGEMENT UNIT
app/view/footer.php
app/view/header.php
CONTACTS DATABASE
app/view/registration_form.php
Add Contact
app/view/search_form.php
Search Contacts
app/view/viewBookings.php
app/Validation.php
{ $valid_type}($value); if (strlen($value)) return $msg; } function time($value) { return ""; } function date($value) { return validateDate($value) ? "" : "Invalid input"; } function alpha_spaces($value) { //只允许空格与字母 $value = str_replace(" ", "", $value); return ctype_alpha($value) ? '' : 'Alpha characters only'; } function digits($value) { //只允许数字 return ctype_digit($value) ? "" : "Digit only"; } function photo($value) { return ""; } function decimal($value) { //验证是否是浮点数 return is_float($value) ? "" : "Float only"; } function email($value) { //验证邮件格式 return filter_var($value, FILTER_VALIDATE_EMAIL) ? "" : "Invaild email"; } function validate_form_data($request, $field) { $errors = []; $validation = new Validation(); foreach ($field as $fieldname => $field_data) { //验证其他: $value = trim($request[$fieldname]); //photo在这里并没有被检测到 $tokens = explode("|", $field_data); $valid_type = $tokens[0]; $require = $tokens[1]; $errorMessage = $this->validate($value, $valid_type, $request); if (strlen($errorMessage) > 0) { $errors[$fieldname] = $errorMessage; } } return $errors; }}
app/myfunctions.php
1 || $numDashes > 1) { $valid = false; } else { // remove spaces $temp = str_replace(" ", "", $value); // remove slash $temp = str_replace("/", "", $temp); // remove dashes $temp = str_replace("-", "", $temp); // check for alpha numeric if (!ctype_alnum($temp)) { $valid = false; } // end if } // end if return $valid;}// end validation of street address/** * Complex names eg Jon-Palo Jnr. the 3rd * Allow alpha numeric, spaces, 1 hyphen, 1 period, 1 digit * @param type $value * @return boolean */function validate_complex_name($value) { $valid = true; // remove the spaces $temp = str_replace(' ', '', $value); // remove dashes and count them $temp = str_replace('-', '', $temp, $hyphen_count); // remove periods and count them $temp = str_replace('.', '', $temp, $fullstop_count); // count the number of digits $digit_count = preg_match_all("/[0-9]/", $temp); // rules are 1 dash, 1 period, 1 digit and alpha permitted if (!ctype_alnum($temp) || $hyphen_count > 1 || $fullstop_count > 1 || $digit_count > 1) { $valid = false; } return $valid;}function validateDOB($value) { $valid = false; if (validateDate($value)) { $today = date('Y-m-d'); $dob = date($value); $time1 = strtotime($today); $time2 = strtotime($dob); if ($time2 < $time1) { $valid = true; } } return $valid;}?>
app/assets/config.php
app/assets/index.php
viewBookings(); } else if ($action == 'addBooking') { $bookingsController->addBooking(); } else if ($action == 'deleteBooking') { $bookingsController->deleteBooking(); } else if ($action == 'editBooking') { $bookingsController->editBooking(); } else if ($action == 'research') { $bookingsController->searchBookings(); } else { include_once "index.php"; }} else { $homeController->index();}include_once 'view/footer.php';
发表评论
最新留言
关于作者
