
Java 操作Excel表格(POI)(Maven),创建表格,画边框线,合并单元格,设置内容,设置行高和列宽,设置对齐方式和字体
发布日期:2021-06-29 15:03:09
浏览次数:3
分类:技术文章
本文共 8768 字,大约阅读时间需要 29 分钟。
Java 操作Excel表格
一、创建表格
1、POI介绍
Apache POI 是用Java编写的免费开源的跨平台的 Java API,Apache POI提供API给Java程式对Microsoft Office格式档案读和写的功能。POI为“Poor Obfuscation Implementation”的首字母缩写,意为“可怜的模糊实现”。
用它可以使用Java读取和创建,修改MS Excel文件.而且,还可以使用Java读取和创建MS Word和MSPowerPoint文件。Apache POI 提供Java操作Excel解决方案(适用于Excel97-2008)结构:
HSSF - 提供读写Microsoft Excel XLS格式档案的功能。 XSSF - 提供读写Microsoft Excel OOXML XLSX格式档案的功能。 HWPF - 提供读写Microsoft Word DOC格式档案的功能。 HSLF - 提供读写Microsoft PowerPoint格式档案的功能。 HDGF - 提供读Microsoft Visio格式档案的功能。 HPBF - 提供读Microsoft Publisher格式档案的功能。 HSMF - 提供读Microsoft Outlook格式档案的功能。2、入门程序
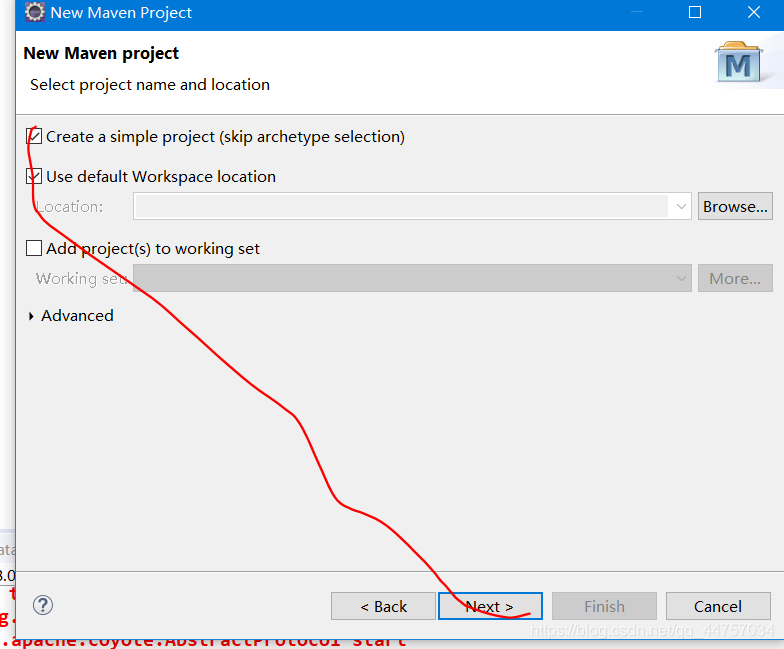
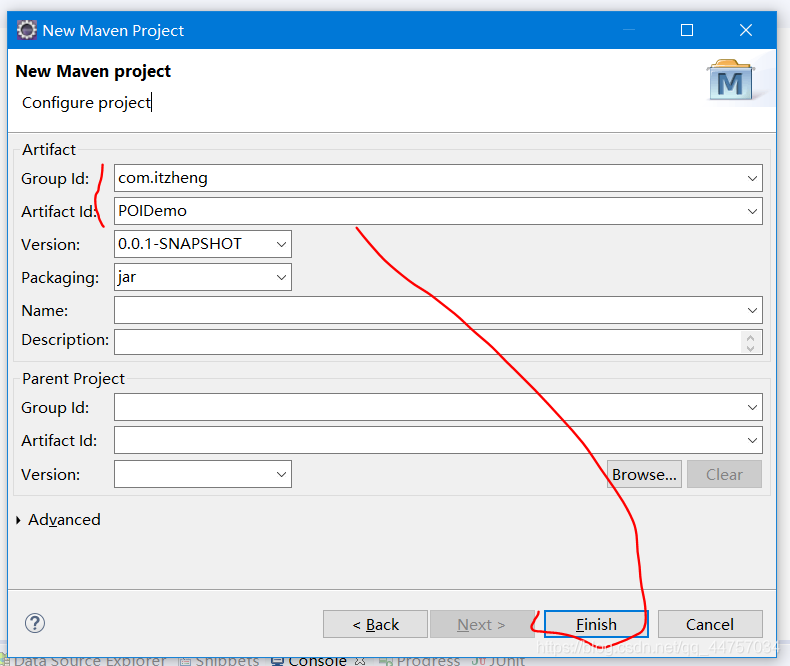
3、添加依赖
4.0.0 com.itzheng POIDemo 0.0.1-SNAPSHOT org.apache.poi poi 3.15
4、创建POIDemo
package com.itzheng.demo.poi;import java.io.File;import java.io.IOException;import org.apache.poi.hssf.usermodel.HSSFCell;import org.apache.poi.hssf.usermodel.HSSFRow;import org.apache.poi.hssf.usermodel.HSSFSheet;import org.apache.poi.hssf.usermodel.HSSFWorkbook;import org.apache.poi.ss.usermodel.Row;import org.apache.poi.ss.usermodel.Workbook;public class POIDemo { public static void main(String[] args) { //创建一个工作簿 HSSFWorkbook wk = new HSSFWorkbook(); //创建工作表 HSSFSheet sheet = wk.createSheet("测试"); //创建一行,行的索引是从0开始 HSSFRow row = sheet.createRow(0); //创建单元格,列的索引是从0 开始 HSSFCell cell = row.createCell(0); //给单元格赋值 cell.setCellValue("测试"); //设置列宽 sheet.setColumnWidth(0, 5000); //width:每个字符的大小,每个字符的大小*256(跟字体有关) File file = new File("D:\\poitest.xls"); try { //保存文件 wk.write(file); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); }finally { try { //关闭流 wk.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }}
运行,创建成功

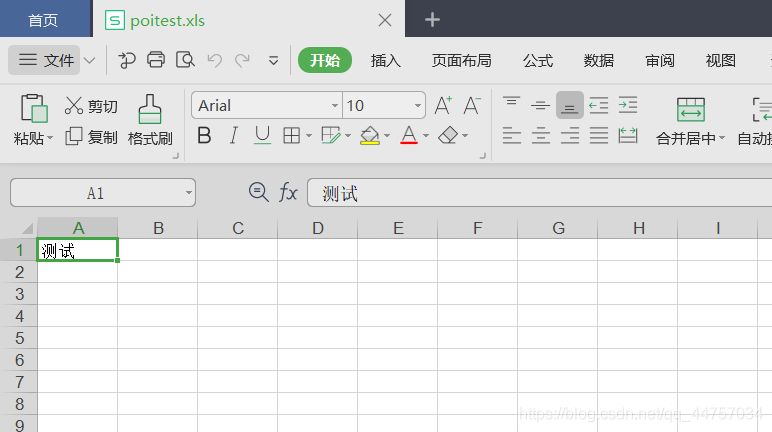
二、特定格式的表格
(一)画边框线
边框线是属于样式范畴,而样式的作用范围是整个工作簿(包括所有的工作表),因此我们可以工作簿来创建样式,再给样式设置边框线
1、创建CreateOrdersTemplate类
package com.itzheng.demo.poi;import java.io.File;import java.io.IOException;import org.apache.poi.hssf.usermodel.HSSFCell;import org.apache.poi.hssf.usermodel.HSSFCellStyle;import org.apache.poi.hssf.usermodel.HSSFRow;import org.apache.poi.hssf.usermodel.HSSFSheet;import org.apache.poi.hssf.usermodel.HSSFWorkbook;import org.apache.poi.ss.usermodel.BorderStyle;/* * 导出的订单模板 */public class CreateOrdersTemplate { public static void main(String[] args) { // 创建一个工作簿 HSSFWorkbook wb = new HSSFWorkbook(); // 创建工作表 HSSFSheet sheet = wb.createSheet("测试"); // 创建一行,行的索引是从0开始 HSSFRow row = sheet.createRow(0); // 创建单元格,列的索引是从0 开始 HSSFCell cell = row.createCell(0); //创建单元格样式 HSSFCellStyle style_content = wb.createCellStyle(); style_content.setBorderBottom(BorderStyle.THIN);//下边框 style_content.setBorderTop(BorderStyle.THIN);//上边框 style_content.setBorderLeft(BorderStyle.THIN);//左边框 style_content.setBorderRight(BorderStyle.THIN);//右边框 //创建11行,4列 for(int i = 2;i <=12;i++) { row = sheet.createRow(i); for (int j = 0; j < 4; j++) { //给单元格设置样式 row.createCell(j).setCellStyle(style_content); } } File file = new File("D:\\ordersTemplate.xls"); try { //保存文件 wb.write(file); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); }finally { try { //关闭流 wb.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }}
(二)合并单元格
//合并单元格 //合并:标题 sheet.addMergedRegion(new CellRangeAddress(0,0,0,3)); //合并第二行 sheet.addMergedRegion(new CellRangeAddress(2,2,1,3)); //合并第7行 sheet.addMergedRegion(new CellRangeAddress(7,7,0,3));
(三)设置内容
//必须先有创建的行和单元格,才可以使用 sheet.getRow(0).getCell(0).setCellValue("采购单"); sheet.getRow(2).getCell(0).setCellValue("供应商"); sheet.getRow(3).getCell(0).setCellValue("下单日期"); sheet.getRow(4).getCell(0).setCellValue("审核日期"); sheet.getRow(5).getCell(0).setCellValue("采购日期"); sheet.getRow(6).getCell(0).setCellValue("入库日期 "); sheet.getRow(3).getCell(2).setCellValue("经办人"); sheet.getRow(4).getCell(2).setCellValue("经办人"); sheet.getRow(5).getCell(2).setCellValue("经办人"); sheet.getRow(6).getCell(2).setCellValue("经办人"); sheet.getRow(7).getCell(0).setCellValue("订单明细");
(四)设置行高和列宽
//设置行高于列宽 //标题行高 sheet.getRow(0).setHeight((short)1000); //内容体的行高 for (int i = 2; i <= 12; i++) { sheet.getRow(i).setHeight((short)500); } //设置列宽 for(int i = 0; i < 4;i++) { sheet.setColumnWidth(i, (short)5000); }
(五)设置对齐方式和字体
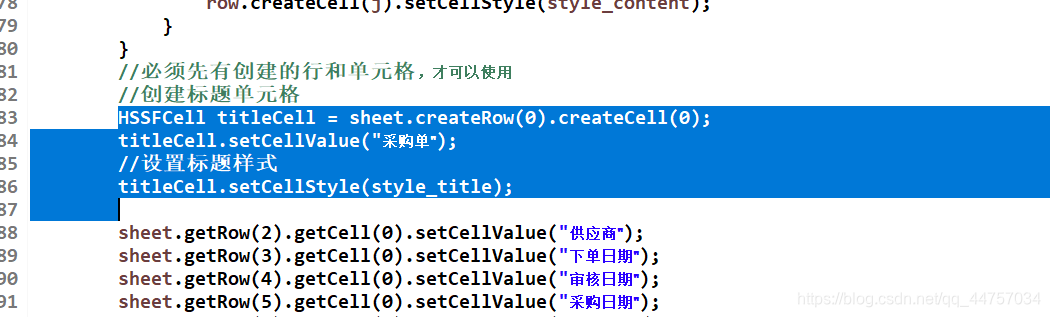
//设置水平对其方式为居中 style_content.setAlignment(HorizontalAlignment.CENTER); //设置垂直对其方式为居中 style_content.setVerticalAlignment(VerticalAlignment.CENTER); //设置标题的样式 HSSFCellStyle style_title = wb.createCellStyle(); style_title.setAlignment(HorizontalAlignment.CENTER); style_title.setVerticalAlignment(VerticalAlignment.CENTER); HSSFFont style_font = wb.createFont(); style_font.setFontName("黑体"); style_font.setFontHeightInPoints((short)18); //加粗 style_font.setBold(true); style_title.setFont(style_font); //创建内容样式的字体 HSSFFont font_content = wb.createFont(); //设置字体名称,相当于选中了那种字符 font_content.setFontName("宋体"); //设置字体的大小 font_content.setFontHeightInPoints((short)11); style_content.setFont(font_content);
全部代码
package com.itzheng.demo.poi;import java.io.File;import java.io.IOException;import org.apache.poi.hssf.usermodel.HSSFCell;import org.apache.poi.hssf.usermodel.HSSFCellStyle;import org.apache.poi.hssf.usermodel.HSSFFont;import org.apache.poi.hssf.usermodel.HSSFRow;import org.apache.poi.hssf.usermodel.HSSFSheet;import org.apache.poi.hssf.usermodel.HSSFWorkbook;import org.apache.poi.ss.usermodel.BorderStyle;import org.apache.poi.ss.usermodel.HorizontalAlignment;import org.apache.poi.ss.usermodel.VerticalAlignment;import org.apache.poi.ss.util.CellRangeAddress;/* * 导出的订单模板 */public class CreateOrdersTemplate { public static void main(String[] args) { // 创建一个工作簿 HSSFWorkbook wb = new HSSFWorkbook(); // 创建工作表 HSSFSheet sheet = wb.createSheet("测试"); // 创建一行,行的索引是从0开始 HSSFRow row = sheet.createRow(0); // 创建单元格,列的索引是从0 开始 HSSFCell cell = row.createCell(0); //创建单元格样式 HSSFCellStyle style_content = wb.createCellStyle(); style_content.setBorderBottom(BorderStyle.THIN);//下边框 style_content.setBorderTop(BorderStyle.THIN);//上边框 style_content.setBorderLeft(BorderStyle.THIN);//左边框 style_content.setBorderRight(BorderStyle.THIN);//右边框 //设置水平对其方式为居中 style_content.setAlignment(HorizontalAlignment.CENTER); //设置垂直对其方式为居中 style_content.setVerticalAlignment(VerticalAlignment.CENTER); //设置标题的样式 HSSFCellStyle style_title = wb.createCellStyle(); style_title.setAlignment(HorizontalAlignment.CENTER); style_title.setVerticalAlignment(VerticalAlignment.CENTER); HSSFFont style_font = wb.createFont(); style_font.setFontName("黑体"); style_font.setFontHeightInPoints((short)18); //加粗 style_font.setBold(true); style_title.setFont(style_font); //创建内容样式的字体 HSSFFont font_content = wb.createFont(); //设置字体名称,相当于选中了那种字符 font_content.setFontName("宋体"); //设置字体的大小 font_content.setFontHeightInPoints((short)11); style_content.setFont(font_content); //合并单元格 //合并:标题 sheet.addMergedRegion(new CellRangeAddress(0,0,0,3)); //合并第二行 sheet.addMergedRegion(new CellRangeAddress(2,2,1,3)); //合并第7行 sheet.addMergedRegion(new CellRangeAddress(7,7,0,3)); //创建11行,4列 //创建矩阵11行,4列 for(int i = 2;i <=12;i++) { row = sheet.createRow(i); for (int j = 0; j < 4; j++) { //给单元格设置样式 row.createCell(j).setCellStyle(style_content); } } //必须先有创建的行和单元格,才可以使用 //创建标题单元格 HSSFCell titleCell = sheet.createRow(0).createCell(0); titleCell.setCellValue("采购单"); //设置标题样式 titleCell.setCellStyle(style_title); sheet.getRow(2).getCell(0).setCellValue("供应商"); sheet.getRow(3).getCell(0).setCellValue("下单日期"); sheet.getRow(4).getCell(0).setCellValue("审核日期"); sheet.getRow(5).getCell(0).setCellValue("采购日期"); sheet.getRow(6).getCell(0).setCellValue("入库日期 "); sheet.getRow(3).getCell(2).setCellValue("经办人"); sheet.getRow(4).getCell(2).setCellValue("经办人"); sheet.getRow(5).getCell(2).setCellValue("经办人"); sheet.getRow(6).getCell(2).setCellValue("经办人"); sheet.getRow(7).getCell(0).setCellValue("订单明细"); //设置行高于列宽 //标题行高 sheet.getRow(0).setHeight((short)1000); //内容体的行高 for (int i = 2; i <= 12; i++) { sheet.getRow(i).setHeight((short)500); } //设置列宽 for(int i = 0; i < 4;i++) { sheet.setColumnWidth(i, (short)5000); } File file = new File("D:\\ordersTemplate.xls"); try { //保存文件 wb.write(file); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); }finally { try { //关闭流 wb.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }}
转载地址:https://code100.blog.csdn.net/article/details/111593842 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
能坚持,总会有不一样的收获!
[***.219.124.196]2024年04月30日 16时05分46秒
关于作者

喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
数据科学中的计量经济学技术
2019-04-29
突破边界:数据科学、数据工程和技术的未来
2019-04-29
一切有迹可循!优秀程序员的9个早期迹象
2019-04-29
在后台的python:众多程序员无法攻克的难题
2019-04-29
未来战争:装载AI的美国空军侦察机已经试飞……
2019-04-29
屡战屡败:为什么你会觉得学习编程很难?
2019-04-29
“狙击”特斯拉:电动汽车后起之秀的最后一战
2019-04-29
软件测试的未来:2021年需要关注的15大软件测试趋势
2019-04-29
六大基本AI术语:如何做好人工智能咨询服务?
2019-04-29
讲真,如果手机有灵魂,那就是“备忘录”
2019-04-29
端到端加密:WhatsApp不会去读取你的信息,它不需要……
2019-04-29
国会大厦骚乱,与一家极不可靠的面部识别公司……
2019-04-29
解锁宇宙密码:为什么是3、6、9?
2019-04-29
数据可视化中的格式塔心理学
2019-04-29
电动汽车的“专属危险”:网络威胁问题不容小觑
2019-04-29
短暂的告别,马上再回来
2019-04-29
统治50年:为什么SQL在如今仍然很重要?
2019-04-29
测试是一场竞争,而数据每次都会获得胜利
2019-04-29
读心的测谎系统:究竟是骗子还是个天才?
2019-04-29
最大规模技术重建:数据库连接从15000个到100个以下
2019-04-29